Integrating ASP. NET Core with Angular is suitable for creating complex and efficient web applications. If these technologies are harnessed properly, you can transform your development environment. This blog covers practices and patterns related to performance, security, capacity, and extensibility.
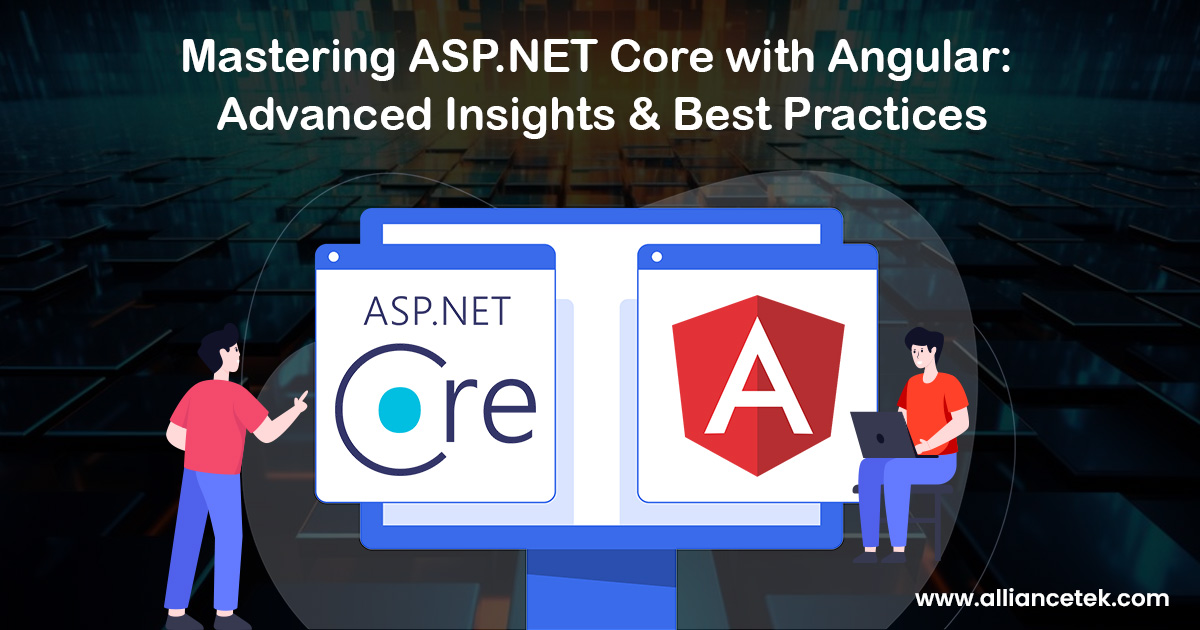
Performance Optimization
# Server-Side-Rendering (SSR)
Server-side Rendering (SSR) is the process of pre-rendering an Angular application on the server. This technique shortens the time to the first meaningful paint, thereby enhancing the user experience . Additionally, SSR delivers content in a format that is easily readable by search engines, improving SEO performance. It is especially useful for highly informational applications with particular emphasis on the interface and rankings.
# Ahead-of-Time (AOT) Compilation
AOT is a feature of Angular used to compile the code in advance, resulting in smaller file sizes of JavaScript and fast rendering. AOT takes the Angular HTML and TypeScript and optimizes it into efficient JavaScript during the build phase, putting less pressure on the browser. This technique improves the application run, thus making the technique suitable for high-performance applications.
# Lazy Loading
The idea of lazy loading implemented in Angular causes modules to be loaded only when required first, improving scalability and reducing application loading time. This is especially helpful in big applications with many modules, controlling the funds allocated to them.
# HTTP/2 Utilization
The major improvements in HTTP/2 are the ability to deliver multiple streams at once and compress headers to speed up data transfer. This leads to faster loading of the web pages and better fluency in the application, especially the ones heavily dependent on APIs.
Security Best Practices
# JWT Authentication
Using JSON Web Tokens (JWT) for stateless authentication enables one to effectively manage the users’ sessions without compromising security . ASP. NET Core can send back JWTs after a successful login. Angular can preserve and send these tokens along with subsequent HTTP requests to check the validity of the generated credentials without relying on the server’s session state.
# CSRF Protection
For protection against CSRF attacks, you can use anti-forgery tokens. Configure Angular’s HttpClient to include these tokens in its HTTP requests, ensuring that only authenticated and authorized requests can modify sensitive data .
# Content Security Policy (CSP)
A strict CSP allows users to prevent XSS with other injection attacks. Set language-specific CSP headers in ASP.NET Core to ensure only code in a specified programming language executes on the site, making it harder for hackers. Configure Angular components to strictly adhere to these policies. This approach creates a strong protective layer against potential vulnerabilities.
# Input Validation and Sanitization
Both client-side (Angular) and server-side (ASP.NET Core) need robust validation mechanisms. While Angular has built-in validators, additional validators from the ASP.NET Core group are essential. ASP.NET Core offers features to protect web applications against injection attacks and validate user data, minimizing data corruption in applications handling sensitive information.
Advanced-Data Handling
Below are some advanced data-handling techniques you can use while using ASP.NET with Angular:
-
Reactive Forms and Observables: Reactive Forms and Observables make it very easy to work with forms and process data asynchronously in Angular. These tools are crucial in designing interfaces for the end users in handling large volumes of data most efficiently.
-
DTOs and AutoMapper: To create DTOs and use AutoMapper in ASP.NET Core, the operating layer is kept separate from the business logic layer. This clean architectural approach simplifies the transformation process and makes the code easier to maintain, especially in data-heavy applications.
-
Server-Side Pagination: Apply server-side pagination in ASP.NET Core APIs to efficiently handle large datasets. This technique reduces the data sent to the client, enhancing load times and memory usage. Angular can then manage the display and navigation of the paginated data.
-
Caching Strategies: Utilizing in-memory and distributed caching strategies in ASP.NET Core can significantly reduce database load and response time due to its asynchronous I/O operations and non-blocking middleware. Additionally, Angular’s HttpClient features enhance client-side performance by caching frequently used data.
Deployment and DevOps
-
CI/CD Pipelines: Configuration of CI/CD pipelines using tools like Azure DevOps, Jenkins, or GitHub Actions helps the implementation of build, testing, and deployment. This automation helps to maintain the periodic release and thereby becomes free from human failures as well as helps to develop the product much faster.
-
Docker Containers: Containerizing your ASP. By using Docker in the NET Core and Angular applications, there is increased compatibility of the environments. Docker containers provide encapsulation of an application and related dependencies and configurations, which makes the process of deployment as well as scaling easier.
-
Kubernetes Orchestration: Kubernetes offers the mechanisms for deploying, scaling, and managing containerized applications when used for container orchestration. Kubernetes offers a highly reliable environment and allows increases or decreases in scale according to the incoming traffic.
-
Monitoring and Logging: Use monitoring and logging solutions like Prometheus/Grafana and ELK Stack (Elasticsearch/Logstash/Kibana). These tools assist in analyzing the performance of an application, finding out problems and even the behavior of users in the application, making management proactive in the process.
Advanced Routing and State Management
# Nested and Lazy-Loaded Routes
The concept of nested configuration is to develop the hierarchical route structure and use the concept of lazy loading to load the route modules during runtime not at the time of application loading. This leads to the improvement of navigational needs in the complexity of the applications developed and maintaining their functionality.
# State Management with NgRx
It is an Angular solution to the state management problem based on the Redux architecture pattern. It guarantees reliable behavior in terms of states and makes code easier to debug. Thus, NgRx is helpful when the application is vast, and there are many dependencies on the state, providing clean state management.
# Global Error Handling
walk through how to implement global error handling with angular’s ErrorHandler and in ASP. NET Core using middleware. Stable error registration is more beneficial for users because the same error codes are used throughout the application, and de-buggin is also easier.
# Breadcrumb Navigation
Introduce the breadcrumb navigation to help users increase the comfort level of their stay within the application by depicting their route at the top of the page. Breadcrumbs aid in finding one’s way in interfaces with many levels and enable one to trace their steps back easily.
Advanced Tooling and Practices
# Install Angular CLI and . NET Core CLI
Angular CLI and .NET Core CLI are both powerful tools, but Angular CLI stands out for its simplicity in usage and setup. It allows you to create components, services, and modules effortlessly. Meanwhile, .NET Core CLI provides rich command-line interfaces that enhance workflow, especially for generating and managing web API projects. These tools automate routine processes, boost efficiency, and ensure adherence to standard development practices.
# Integrating Third-Party Libraries
Implementation of third-party libraries may indeed act as one of the most helpful sources to develop more functionality and save much time. For example, compatibility with Angular Material opens a series of predefined user interface elements that follow Google’s material design specifications. Likewise, while applying some libraries such as AutoMapper in ASP. NET Core also has functions for data mapping and transforming.
# Testing and Quality Assurance
It is especially important when creating pieces of software to follow an appropriate set of strategies aimed at testing the results. For the AngularJS applications, unit tests can be made through tools like Jasmine and Karma while xUnit for ASP. NET Core tests make significant assurance that both the frontend as well as backend are well tested. Continuously integration of test cases also makes it easier in the CI/CD pipelines and reduces the chances of regression.
# Code Reviews and Pair Programming
Thus, the authors have identified that normal code reviews and pair programming can go a long way in enhancing the code quality while enhancing the spread of information among the development team. It allows the detection of some problems in advance, assessing the completion of coding standards, and the openness of the development process.
# Future-Proofing Your Applications
Both Angular and ASP. All NET Core are alive and updated frequently. Updating your applications to use the current version of these frameworks allows you to take advantage of improved performance, newly available features, and security corrections. Subscribing to release notes and integrating changes in the development process will keep applications safe from obsolescence.
# Adopting Progressive Web App (PWA) Standards
PWAs can be described as the best of both worlds of web and mobile applications. You can get your angular applications privileged with offline support, push notifications, and optimizing for mobile with the help of PWA standards. ASP. ASP. These features can easily be implemented in the APIs that are used in NET Core, hence acting as a strong back end for PWAs.
# Exploring Microservices Architecture
Microservices help increase the scalability, maintainability, and resiliency of the application by splitting up your application into several independent deployable services. ASP. Hence, it is easily possible for NET Core to support microservices and Angular as it has a modular structure to support such an architecture properly.
Conclusion
Integrating ASP.NET Core with Angular is not just about combining two powerful technologies—it's about leveraging their strengths to create powerful, scalable web applications. By focusing on performance optimization, security best practices, efficient data handling, robust deployment strategies, and advanced routing and state management techniques, you can develop applications that are not only performant but also secure and maintainable. This deep integration leverages the best aspects of both frameworks, providing a comprehensive solution for modern web development challenges.
Call us at 484-892-5713 or Contact Us today to know more details about Mastering ASP.NET Core with Angular: Advanced Insights and Best Practices.