When it comes to software development, there are different approaches and tools that can be used to achieve the desired functionality. Two commonly used methods are stored procedures and LINQ. Both have their advantages and disadvantages, and the choice between them depends on several factors that need to be considered during the decision-making process.
The size and scope of your development project are important factors to consider when deciding whether to use stored procedures or LINQ. The choice between stored procedures and LINQ depends on several factors, including the size and scope of your development project, the skills of your coders, and your hardware. It's important to consider all of these factors when making a decision to ensure that you choose the best option for your specific needs.
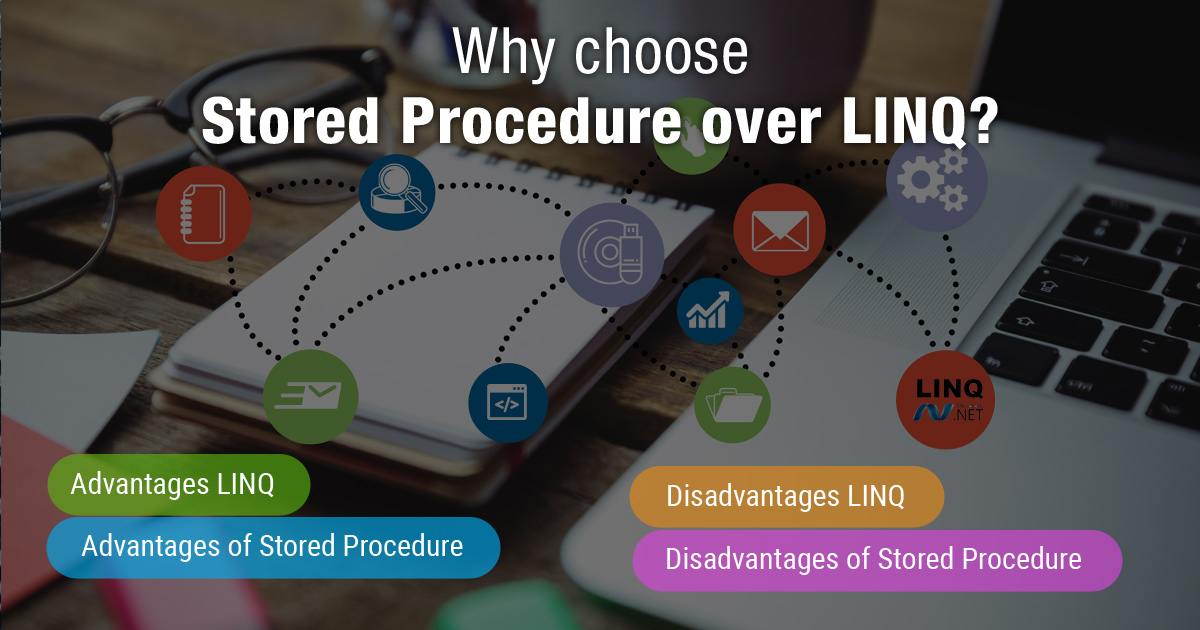
Understanding LINQ
LINQ (Language-Integrated Query) is a versatile and flexible technology that allows developers to query data from various sources in a concise and expressive manner. The term "Language-Integrated" refers to the fact that LINQ is built into the C# and Visual Basic programming languages, which means that developers can write queries using the familiar syntax of these languages.
One of the key benefits of LINQ is that it enables developers to perform complex queries and transformations on data with ease. Whether querying databases, XML documents, or in-memory collections, LINQ provides a unified approach that eliminates the need for developers to learn different query languages for each data source.
Additionally, LINQ is well-suited for projects that require a minimal amount of boilerplate code. When a LINQ query is executed, it is compiled just in time, which means that developers don't need to write separate code to interact with the database or other data sources. This makes LINQ a popular choice for rapid application development services and prototyping.
Another advantage of LINQ is its expressive and lucid syntax, which allows developers to write powerful queries with fewer lines of code. This makes code easier to read and maintain, and can also improve performance by reducing the amount of work that the compiler needs to do.
Understanding Stored Procedure
A stored procedure is a group of pre-written SQL statements that are compiled and stored in a database. They are used to perform a specific task or a set of tasks in a database. Stored procedures are often used to perform complex operations such as data transformation, validation, and aggregation.
One of the main advantages of using stored procedures is that they offer better performance and scalability than traditional SQL statements. This is because stored procedures are compiled and stored in compiled form in the database, which means that they can be executed more quickly than a series of ad-hoc SQL statements. Additionally, because stored procedures are cached, they can be reused multiple times, which reduces the need for repetitive SQL code.
Stored procedures are also well-suited for long-term projects because they are written once and can be shared between different programs or applications. This means that if a particular task or operation needs to be performed across multiple programs or applications, the same stored procedure can be used in each instance. This can help to reduce the amount of code duplication, improve code reuse, and make it easier to maintain and update the codebase over time.
Another advantage of using stored procedures is that they can help to improve security in a database. Because stored procedures are compiled and stored in the database, they can be granted specific permissions and access controls. This means that access to sensitive data or operations can be restricted to authorized users only, which can help to prevent unauthorized access and data breaches.
Advantages of LINQ
- LINQ is tightly integrated with strongly typed data, which means that it allows developers to access table columns as a property. This makes it easier to work with data and reduces the likelihood of errors or bugs caused by type mismatches.
- Because LINQ is type-safe, query errors are type checked at compile time. This helps to catch errors early on in the development process and can save time and effort that would otherwise be spent debugging.
- LINQ is a universal query language that can be used to work with a wide range of data sources, including databases, XML files, JSON files, lists, arrays, and dictionaries. This makes it a powerful and versatile tool for developers and eliminates the need to learn and use multiple query languages for different data sources.
- LINQ is easier to debug with the use of visual studio's debugger through .Net Development Solutions. This makes it easier for developers to identify and fix errors in their code and can help to reduce the time and effort required to debug complex queries or data transformations.
Advantages of Stored Procedure
- SQL queries are entered in a simple language that is easy to understand and use. This means that dedicated developers with little or no experience in SQL can quickly learn how to write and execute queries.
- They offer directly operative features such as encryption and decryption of data at the column level. This means that data can be protected by encrypting sensitive data, and decrypted only when necessary.
- SQL queries also offer the ability to cache queries, which can lead to better performance. When a query is executed, the database server stores the results in cache memory. This can help to improve the speed of data retrieval and reduce the time needed to execute complex queries.
- They can be used to join data from multiple tables, aggregate data, and perform complex calculations and transformations.
Disadvantages of LINQ
- LINQ statements provide a powerful way to manipulate data in C# and Visual Basic. While the syntax of LINQ statements may seem compact on the surface, complex queries are actually created under the hood.
- LINQ statements process a complete query each time it executes, which can impede performance. This means that even if only a small amount of data has changed since the last query was executed, the entire query will still be reprocessed.
- They do not directly support features like encryption and decryption. While it is possible to encrypt and decrypt data using LINQ statements, it is typically done using other tools and techniques. This can add complexity and increase the likelihood of errors or security vulnerabilities.
Disadvantages of Stored Procedure
- SQL queries and stored procedures can become quite complex, with many nested statements, conditions, and functions. This complexity can make it difficult to identify and fix bugs or errors and can make maintenance and updates time-consuming and labor-intensive.
- SQL queries and stored procedures are often specific to a particular version of a database or database management system. This means that when upgrading to a new version of the same database, or when migrating to a different database system altogether, developers may need to spend a significant amount of time rewriting or modifying their code.
- Database code can also be resource-intensive. Queries and stored procedures can utilize database server resources such as memory, CPU, and I/O. This can impact the performance and responsiveness of the database system, especially when working with large data sets or complex queries.
Factors That Determine Using Stored Procedures or LINQ
# Performance Optimization
Stored procedures are a good choice for large projects that involve complex queries and require significant performance optimization. Stored procedures are pre-compiled and stored on the database server, which can result in faster execution times compared to LINQ. Additionally, stored procedures can be used to encapsulate business logic and improve data security by limiting direct access to the database. On the other hand, LINQ is a better choice for smaller projects that require simple queries and don't need a lot of performance optimization.
# Coder Skills
The skills of your coders are another factor to consider when deciding between stored procedures and LINQ. Stored procedures require a good understanding of SQL and database architecture, as well as knowledge of the specific database management system being used. In contrast, LINQ is a more developer-friendly option that doesn't require as much specialized knowledge of database systems. Developers who are proficient in C# or other PHP .Net Development Solutions languages can easily write LINQ queries using object-oriented programming concepts.
# Hardware
Your hardware is also an important factor to consider when deciding between stored procedures and LINQ. Stored procedures can be more resource-intensive than LINQ because they are executed on the database server, which requires additional server resources. If you have a limited hardware budget, LINQ may be a better choice because it places less strain on the server.
When to Choose Stored Procedure Over LINQ
Stored procedures are particularly well-suited for projects with complex data requirements, as they allow for more efficient data retrieval and processing. However, stored procedures can also be more difficult to maintain and debug, as they are typically written in SQL and can be quite complex.
LINQ, on the other hand, is a more user-friendly approach to working with data. LINQ provides a simple, intuitive syntax for manipulating data in C# and Visual Basic, which can make development faster and easier. LINQ is particularly well-suited for projects that require basic CRUD (create, read, update, delete) operations, as it allows for rapid development and easy maintenance. However, LINQ may not be powerful enough for more complex projects over time, as it can be less efficient than stored procedures and may not provide the same level of performance.
Ultimately, the choice between stored procedures and LINQ depends on the specific needs and requirements of the project. For projects with complex data requirements, stored procedures may be the better choice, as they offer enhanced performance and scalability. However, for projects with simpler data requirements, LINQ may be a more user-friendly and efficient option. In any case, it's important for developers to carefully consider the pros and cons of each approach before making a decision, in order to ensure that the project meets its goals and objectives.
Call us at 484-892-5713 or Contact Us today to know more details about the Why Choose Stored Procedure over LINQ?.