Web developers are used to using the popular API XMLHttpRequest to send HTTP or HTTPS requests directly to a web server and load the server response data directly back to the script. XMLHttpRequest has an especially important role in Ajax web development where it is often used to implement responsive and dynamic web applications, such as Gmail, Google Maps, and Facebook.
Unfortunately, one troubling aspect of working with most browsers is using cross-domain requests involving two domains. On these browsers, XMLHttpRequests that use two domains are prohibited due to a security restriction called “same origin policy.” For example, if you are running a web application on appdomain.com and want to invoke an XMLHttpRequest on appdomain2.com, this would be prohibited.
According to this security policy, browsers prevent a Request loaded from one origin from getting or setting properties of a document from another origin. Mozilla has been using this security policy all the way back to Netscape Navigator 2.0, so it’s not likely to change soon.
Fortunately, there are a few ways to circumvent the “same origin policy” regarding cross-domain script loading:
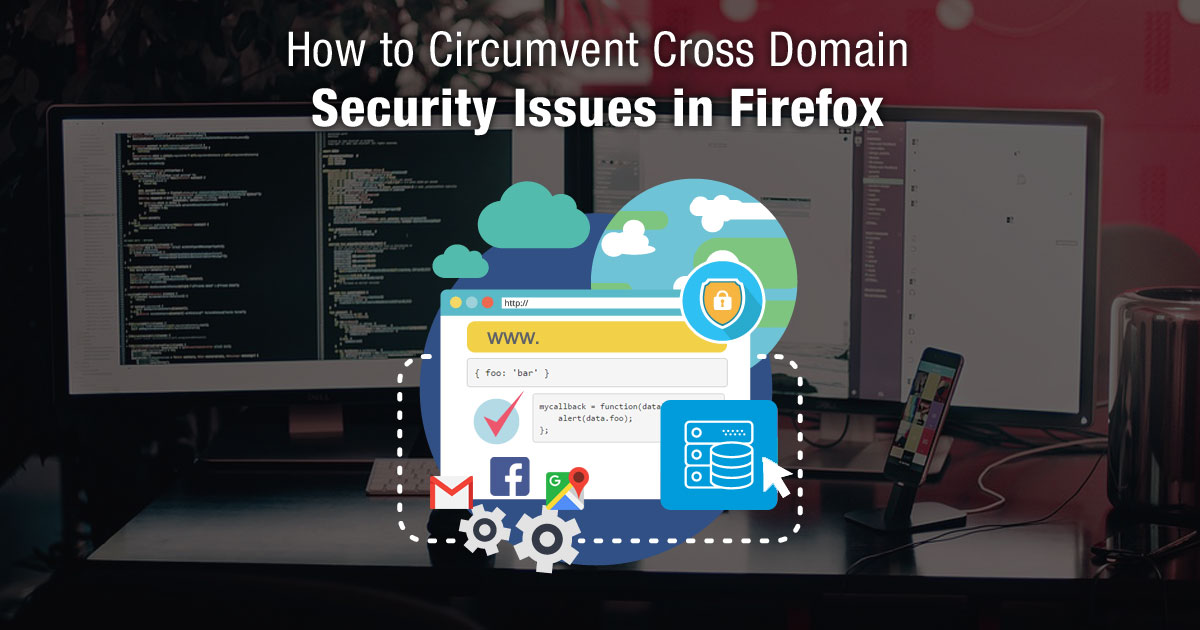
Introducing a Proxy Server
A proxy server that is located on the same domain as the client accepts a request directed to another domain.
However, the proxy server makes a request to such a target external domain. Since this request originates from one server to another, it is not affected by the same-origin policy enforced in browsers.
When the proxy server receives a response from a target server, it returns this information to the user. To the client, it seems to be an answer from a single domain which avoids cross-domain issues.
# Practical Usage and Benefits
Proxy servers are useful in scenarios where data from various external APIs needs to be aggregated. The server can collect this data and present it as a unified response to the client.
By using a proxy server, developers can implement additional security measures, like filtering requests and responses or adding authentication layers that the client might not directly handle.
Proxy servers can cache responses, thereby improving response times for subsequent requests for the same resources. This can significantly enhance the performance of web applications.
# Implementing a Proxy Server
Implementation involves setting up a server-side script or program that handles incoming requests, makes external requests as needed, and returns the results. This can be done in various server environments like Node.js, Apache, or Nginx.
The server can be configured to handle specific types of requests, cache data, manage session persistence, and even load balance between multiple external sources.
# Considerations and Drawbacks
Introducing an additional layer in the request-response cycle can add latency. This is particularly relevant if the proxy server experiences high traffic or is not optimally configured.
Running a proxy server requires additional maintenance. Ensuring its security is crucial since it handles incoming and outgoing data that might be sensitive.
Setting up a proxy server can be complex, especially in distributed systems where scaling becomes a factor. It requires careful planning and resources to manage effectively.
JSONP
Even though browsers are reluctant to make cross-domain Ajax calls, another way to bypass them is by using <script> tags. With script tags (such as <script>, <img>, or <iframe>), the domain limitation is ignored, although you can’t do anything with the result as it is simply evaluated. However, by using JSONP you can pass a special parameter that gives the server some information about the page. So you have the server create a response in a way that your page can handle.
For example, a server expects a parameter called “callback” to enable its JSON capabilities. The Request could be:
- http://www.xyz.com/sample.aspx?callback=mycallback
Ordinarily, the server might return some basic JavaScript, like:
{ foo: 'bar' }
But with JSON, the server can use the “callback” parameter to change the result to something like:
mycallback({ foo: 'bar' });
This will not invoke the method you specified. Your page can define the callback function:
mycallback = function(data)
alert(data.foo);
};
When loaded, the script will be evaluated, and the function executed. A third-party JavaScript library like jQuery can achieve the above functionality:
$.ajax({
url: “http://www.xyzdomain.com/test.aspx”,
dataType: “jsonp”,
success: function(data){
alert(data);
}
});
CORS
The CORS is a new specification introduced by W3C which will allow cross-domain JavaScript requests that would have been blocked due to the same origin policies.
According to CORS specifications, the caller will send a request by adding an “Origin” parameter (into the request header) to the request. Suppose the request is approved by the remote endpoint. In that case, the caller will then receive a response that includes a particular parameter that denotes the success of the request called “Access-Control-Allow-Origin” and is sent to any site to access the body of the HTTP response.
By 2024, CORS has become widely adopted and is an essential part of web development. Its implementation now extends to a wider array of web applications, catering to increasingly dynamic and interactive online environments. This expansion is critical in an era where applications are no longer isolated but interconnected across various domains.
ORS has evolved to support more sophisticated functionalities, bridging gaps between different web services and APIs. This is particularly significant for applications that rely on pulling data from multiple sources, such as integrated social media feeds, data from various APIs in single-page applications (SPAs), and real-time data streaming. CORS facilitates these functionalities by enabling secure and controlled access to resources across different origins.
Practical Implementation Examples
# Scenario-Based Implementation
- In a basic blog application, CORS can be implemented to allow the fetching of posts from a different domain API. Here, the server might include headers like Access-Control-Allow-Origin: https://blogdomain.com to permit requests from the blog's domain.
- An e-commerce site might integrate reviews from a third-party service. CORS settings on the review service’s server would include specific headers allowing requests from the e-commerce domain, and potentially specify which HTTP methods are permissible, ensuring a secure data exchange.
- For a dashboard aggregating data from multiple sources, the server might need to handle more complex CORS requests. Here, preflight requests would be common, especially if custom headers are used for API keys or authentication tokens.
# Setting Up CORS Headers
- Developers can configure their servers to respond with appropriate CORS headers. For example, in a Node.js environment, using middleware like CORS in Express.js can simplify this process.
- Set up the server to handle preflight requests by responding appropriately in OPTIONS requests. This covers checking for Access-Control-Request-Method and Access-Control -Request Headers, responding with the matching *Access Control Allow Header.
Additional Methods to Circumvent Cross-Domain Issues
# WebSockets for Cross-Domain Communication
WebSockets offer full-duplex mode of communication which allows real time, end to end bidirectional connectivity between the client and server. This is a step up from the traditional request-response model used by XMLHttpRequest.
Unlike traditional methods restricted by same-origin policies, WebSockets can connect to any server, irrespective of the domain. This makes them ideal for situations where live data needs to be streamed from a different domain.
They are widely used in applications requiring real-time data exchange, like chat applications, live sports updates, and financial tickers.
Setting up a WebSocket involves creating a WebSocket server and connecting to it using the WebSocket API in JavaScript, typically initiated with a new WebSocket(URL).
# Post Message for Secure Cross-Domain Messaging
- The window.postMessage() method allows secure cross-origin communication between Window objects; for example, between a page and a pop-up it spawned, or between a page and an iframe embedded within it.
- It enables the sending of data across origins while ensuring the recipient is the intended one, thus maintaining security. The recipient window can listen to the data using an event listener.
- Developers have control over what data is sent and can implement validation to ensure that the communication is secure and restricted to trusted domains only.
# Server-Side Proxying
In server-side proxying, a server on the same domain as the client acts as an intermediary. This server receives requests from the client and passes them to the external domain.
The method circumvents same-origin policy restrictions since, from the client's point of view, all requests are sent to and received only by a single one.
Using the Document.domain Property
- Adjusting the document.domain property can enable communication between different pages across subdomains. By setting this property to a common domain, pages from different subdomains can interact as if they were from the same origin.
- This method is only applicable to pages that have the same second-level domain. For example, sub1.example.com and sub2.example.com can communicate if they both set document.domain to example.com.
- It's a simpler solution compared to others, particularly useful when dealing with iframe content or scripts loaded from subdomains.
Call us at 484-892-5713 or Contact Us today to know more details about how to circumvent cross-domain security issues in Firefox.